The final requirement to implement the CheckingAccount
class is to define a constructor, whose purpose is to initialize the values of instance variables of an object. To construct objects of the CheckingAccount
class, it is necessary to declare an object variable first.
CheckingAccount checking;
Object variables such as checking
are references to objects. Instead of holding an object itself, a reference variable holds the information necessary to find the object in memory. This is the address of the object.
- The object identifier
checking
does not refer to any object yet. An attempt to invoke a method on this variable would cause a runtime null pointer exception error. To initialize the variable, it is necessary to create a new CheckingAccount
object using the new
operator
checking = new CheckingAccount();
Constructors are always invoked using the new
operator. The new
operator allocates memory for the objects, and the constructor initializes it. The “new” operator returns the reference to the newly constructed object.
In most cases, you will declare and store a reference to an object in an object identifier on one line as follows:
CheckingAccount checking = new CheckingAccount();
Occasionally, it would be repetitive and unnecessary to create an object identifier. If the purpose of creating the object is only to pass it in as an argument, you can simply create the object within the method call. For example, when creating DrawingTool
objects, and you are providing a SketchPad
object, you do not need to create an identifier for that SketchPad
object:
DrawingTool pen = new DrawingTool(new SketchPad(500,500));
Notice that we never create an object identifier for the SketchPad object.
Constructors always have the same name as their class. Similar to methods, constructors are generally declared as public to enable any code in a program to construct new objects of the class. Unlike methods, constructors do not have return types.
-
Instance variables are automatically initialized with a default value (0 for number, false for boolean, null for objects). Even though initialization is handled automatically for instance variables, it is a matter of good style to initialize all instance variables explicitly. Generally, all of your instance variables should be initialized in your constructor.
- Many classes define more than one constructor through overloading. For example, you can supply a second constructor for the CheckingAccount class that sets the myBalance and myAccountNumber instance variables to initial values, which are the parameters of the constructor:
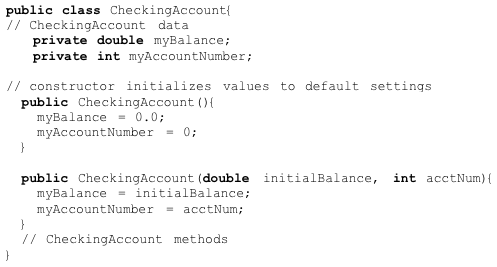
The second constructor is used if you supply a starting balance and an account number as construction parameters.
CheckingAccount checking = new CheckingAccount(5000.0, 12345);
The number of constructors is based on the needs of the client.